To search for all browsers installed on a Windows system using PowerShell, you can utilize the Get-ItemProperty cmdlet. This cmdlet allows you to access classes, which provide information about various aspects of the operating system.
To start, open PowerShell by typing “PowerShell” in the Windows search bar and selecting the Windows PowerShell app. Once the PowerShell window is open, you can begin by running the following command:
“`
$uninstallKeys = ‘HKLM:\Software\Microsoft\Windows\CurrentVersion\Uninstall*’, ‘HKLM:\SOFTWARE\Wow6432Node\Microsoft\Windows\CurrentVersion\Uninstall*’
$softwareList = Get-ItemProperty -Path $uninstallKeys |
Select-Object DisplayName, Publisher, InstallDate, DisplayVersion |
Where-Object { $_.DisplayName -match ‘Chrome|Firefox|Edge|Opera’ }
$softwareList
“`
Here is the output of the command in PowerShell:
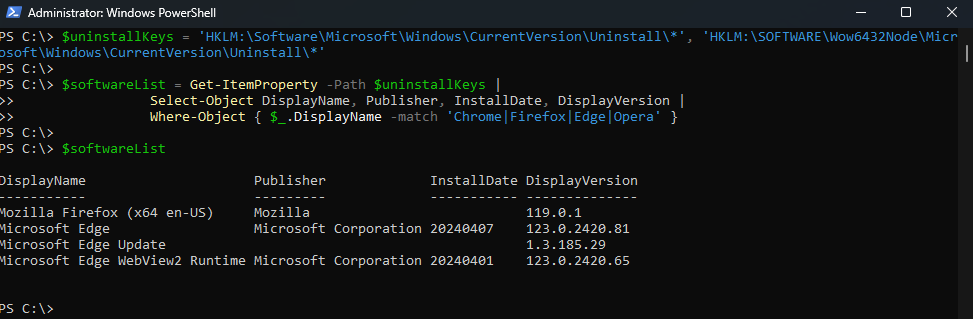
This command uses the Get-ItemProperty cmdlet to retrieve information from the Win32_Product class, which contains details about installed software on the system. The Where-Object cmdlet is then used to filter the results based on the browser names. In this example, the command searches for browsers with names containing “Chrome,” “Firefox,” “Edge,” or “Opera.”
When you run the command, PowerShell will display a list of all the browsers that match the specified criteria. The output will include information such as the name, version, vendor, and installation location of each browser.
Using PowerShell to search for installed browsers can be particularly useful in scenarios where you need to gather information about the software installed on multiple machines in a network. By running the command remotely or through a script, you can quickly obtain a comprehensive list of browsers across multiple systems.
In addition to searching for specific browser names, you can also modify the command to retrieve information about other software installed on the system. The Win32_Product class contains details about various applications, not just browsers. You can adjust the filter criteria in the Where-Object cmdlet to search for different software based on your requirements.
Overall, PowerShell provides a powerful and flexible way to search for browsers and other software installed on a Windows system. By leveraging the capabilities of PowerShell, system administrators and curious users can easily gather information about the software landscape on their machines or across a network.
Using PowerShell to Search for Installed Browsers
To begin, open PowerShell on your Windows machine. You can do this by pressing the Windows key, typing “PowerShell,” and selecting the “Windows PowerShell” option.
Once PowerShell is open, you can use the following command to search for installed browsers:
Get-ItemProperty 'HKLM:\Software\Microsoft\Windows\CurrentVersion\App Paths\*.exe' | Where-Object { $_.PSChildName -match "chrome|firefox|edge|opera" } | Select-Object -Property PSChildName
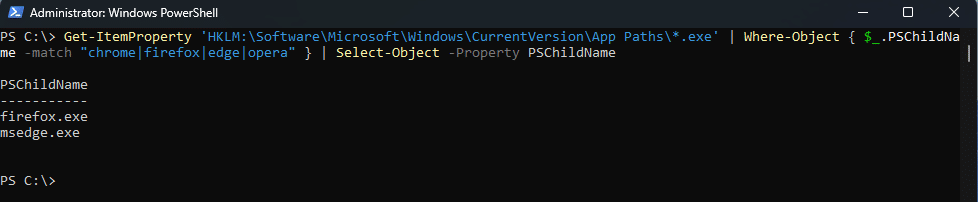
This command retrieves the properties of items in the Windows Registry that match the specified pattern. In this case, we are searching for any executable files associated with popular browsers such as Chrome, Firefox, Edge, and Opera.
The output of this command will display the names of the executable files for each installed browser. For example, you might see something like:
This indicates that Google Chrome, Mozilla Firefox, Microsoft Edge, and Opera are installed on the system.
PSChildName
-----------
chrome.exe
firefox.exe
msedge.exe
opera.exe
Once you have identified the installed browsers, you can use PowerShell to perform various tasks related to these browsers. For example, you can use PowerShell to automate the opening of a specific browser and navigate to a specific website. This can be useful if you need to perform repetitive tasks or if you want to create a script that interacts with a specific browser.
In addition to automating browser tasks, PowerShell can also be used to manage browser settings. For example, you can use PowerShell to change the homepage or default search engine of a browser. This can be helpful if you want to enforce certain settings across multiple machines or if you want to streamline the configuration process for new installations.
Furthermore, PowerShell can be used to gather information about browser usage on a system. You can use PowerShell to retrieve data such as the version number of a browser, the number of tabs currently open, or the browsing history of a user. This information can be useful for troubleshooting purposes or for monitoring user activity.
Overall, PowerShell provides a powerful and flexible tool for managing and interacting with installed browsers on a Windows machine. Whether you need to automate tasks, manage settings, or gather information, PowerShell can help streamline your browser-related workflows and improve productivity.
Understanding the PowerShell Command
Let’s break down the PowerShell command we used to search for installed browsers:
Get-ItemProperty HKLM:SoftwareMicrosoftWindowsCurrentVersionApp Paths\*.exe
This part of the command retrieves the properties of items in the Windows Registry. The specified path, “HKLM:SoftwareMicrosoftWindowsCurrentVersionApp Paths**.exe,” represents the registry key where information about installed applications is stored. The “Get-ItemProperty” cmdlet allows us to access and retrieve the properties of the items in this registry key.
Where-Object { $_.PSChildName -match "chrome|firefox|edge|opera" }
This section filters the results based on the PSChildName property, which represents the name of the executable file. The “-match” operator is used to match the PSChildName against a regular expression pattern that includes the names of popular browsers. By using the “Where-Object” cmdlet, we can specify a condition that the PSChildName must meet in order to be included in the final results. In this case, the condition is that the PSChildName must match any of the specified browser names.
Select-Object -Property PSChildName
Finally, the “Select-Object” cmdlet is used to select and display only the PSChildName property, which corresponds to the names of the installed browsers. By using the “Select-Object” cmdlet, we can choose specific properties from the objects returned by the previous cmdlets and display only those properties. In this case, we are interested in displaying the PSChildName property, which represents the names of the installed browsers.
Now that we have broken down the PowerShell command and understood each component, let’s take a closer look at how it works in practice. When we execute this command, PowerShell will first access the specified registry key and retrieve the properties of all the items within it. This includes the PSChildName property, which represents the name of the executable file for each installed application.
Next, the command applies a filter using the “Where-Object” cmdlet. This filter checks if the PSChildName matches any of the specified browser names: “chrome”, “firefox”, “edge”, or “opera”. If a match is found, the item is included in the final results; otherwise, it is excluded.
Finally, the “Select-Object” cmdlet is used to display only the PSChildName property for the filtered items. This ensures that we only see the names of the installed browsers in the output.
By breaking down and understanding the individual components of this PowerShell command, we can see how it allows us to efficiently search for and retrieve information about installed browsers on a Windows system. This knowledge can be valuable for various purposes, such as troubleshooting browser-related issues or performing system audits.
Customizing the PowerShell Command
If you want to search for additional browsers or modify the command to suit your specific needs, you can edit the regular expression pattern in the “Where-Object” section of the command.
For example, if you want to search for the Brave browser as well, you can modify the command like this:
Get-ItemProperty 'HKLM:SoftwareMicrosoftWindowsCurrentVersionApp Paths\*.exe' | Where-Object { $_.PSChildName -match "chrome|firefox|edge|opera|brave" } | Select-Object -Property PSChildName
This modified command includes the name “brave” in the regular expression pattern, allowing it to match the executable file of the Brave browser.
By customizing the regular expression pattern, you can search for any browser executable you desire. For instance, if you want to include Safari in the search, you can simply add “safari” to the regular expression pattern, like this:
Get-ItemProperty 'HKLM:SoftwareMicrosoftWindowsCurrentVersionApp Paths\*.exe' | Where-Object { $_.PSChildName -match "chrome|firefox|edge|opera|brave|safari" } | Select-Object -Property PSChildName
This will now include Safari in the search and provide you with the executable file name if it is found.
Feel free to experiment and customize the command further to meet your specific requirements. You can add or remove browser names from the regular expression pattern as needed, allowing you to search for any combination of browsers that you want.
Additionally, you can modify other parts of the command to suit your needs. For example, you can change the property you want to select by modifying the “Select-Object” section. Instead of selecting the “PSChildName” property, you can select other properties such as “Version” or “Path”.
Remember to test your modified command to ensure it provides the desired results. PowerShell is a powerful tool that allows you to customize commands to fit your specific requirements, giving you flexibility and control over your search for browser executables.
Hope you find it helpful.